Animation
Animation:
Animation in the objective of Android means the process of making movement and other graphic effects in order to enrich the interface of the sociable application and, therefore, improve the overall usage experience.
Some of them include: view, images, and graphics in which the way they are moved from one place to the other or its movement creates a smooth or an intended effect.
Example:
<!-- activity_main.xml -->
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<ImageView
android:id="@+id/imageview"
android:layout_width="80dp"
android:layout_height="80dp"
android:layout_centerHorizontal="true"
android:layout_marginTop="40dp"
android:contentDescription="@string/app_name"
android:src="@drawable/ic_smartphone" />
<ScrollView
android:layout_below="@id/imageview"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_marginTop="10dp"
android:scrollbars="none">
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_alignParentStart="true"
android:layout_alignParentEnd="true"
android:layout_alignParentBottom="true">
<!-- Buttons for different animations -->
<LinearLayout
android:id="@+id/linear1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
android:orientation="horizontal"
android:weightSum="3">
<Button
android:id="@+id/blink"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_margin="3dp"
android:layout_weight="1"
android:text="BLINK"
android:textColor="#FFFFFF"
android:background="#311b92" />
<Button
android:id="@+id/slide_up"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_margin="3dp"
android:layout_weight="1"
android:text="SLIDE UP"
android:textColor="#FFFFFF"
android:background="#311b92" />
<Button
android:id="@+id/slide_down"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_margin="3dp"
android:layout_weight="1"
android:text="SLIDE DOWN"
android:textColor="#FFFFFF"
android:background="#311b92" />
</LinearLayout>
<!-- More Buttons for different animations -->
<!-- Stop Button -->
<Button
android:id="@+id/stop"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/linear4"
android:layout_marginLeft="30dp"
android:layout_marginTop="10dp"
android:layout_marginRight="30dp"
android:layout_marginBottom="70dp"
android:text="Stop"
android:textColor="#FFFFFF"
android:background="#E65100"/>
</RelativeLayout>
</ScrollView>
</RelativeLayout>
<!-- blink_animation.xml -->
<set xmlns:android="http://schemas.android.com/apk/res/android">
<alpha
android:fromAlpha="0.0"
android:toAlpha="1.0"
android:interpolator="@android:anim/accelerate_interpolator"
android:duration="1000"
android:repeatMode="reverse"
android:repeatCount="infinite"/>
</set>
<!-- slideup_animation.xml -->
<set xmlns:android="http://schemas.android.com/apk/res/android" >
<translate
android:duration="1000"
android:fromYDelta="0"
android:toYDelta="-100%" />
</set>
<!-- slidedown_animation.xml -->
<set xmlns:android="http://schemas.android.com/apk/res/android" >
<translate
android:duration="1000"
android:fromYDelta="0"
android:toYDelta="100%" />
</set>
<!-- rotate_animation.xml -->
<set xmlns:android="http://schemas.android.com/apk/res/android">
<rotate
android:duration="1000"
android:fromDegrees="0"
android:pivotX="50%"
android:pivotY="50%"
android:toDegrees="360" />
<rotate
android:duration="1000"
android:fromDegrees="360"
android:pivotX="50%"
android:pivotY="50%"
android:startOffset="5000"
android:toDegrees="0" />
</set>
<!-- zoomin_animation.xml -->
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:fillAfter="true" >
<scale
android:duration="2500"
android:fromXScale="1.0"
android:fromYScale="1.0"
android:pivotX="50%"
android:pivotY="50%"
android:toXScale="3.0"
android:toYScale="3.0" >
</scale>
</set>
<!-- zoomout_animation.xml -->
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:fillAfter="true" >
<scale
android:duration="2500"
android:fromXScale="1.0"
android:fromYScale="1.0"
android:pivotX="50%"
android:pivotY="80%"
android:toXScale="0.2"
android:toYScale="0.2" >
</scale>
</set>
<!-- crossfade_animation.xml -->
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:fillAfter="true" >
<alpha
android:duration="800"
android:fromAlpha="0.0"
android:interpolator="@android:anim/accelerate_interpolator"
android:toAlpha="1.0" />
<alpha
android:duration="1100"
android:fromAlpha="1.0"
android:interpolator="@android:anim/accelerate_interpolator"
android:toAlpha="0.0" />
</set>
<!-- fadein_animation.xml -->
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:fillAfter="true" >
<alpha
android:duration="1100"
android:fromAlpha="0.0"
android:interpolator="@android:anim/accelerate_interpolator"
android:toAlpha="1.0" />
</set>
<!-- fadeout_animation.xml -->
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:fillAfter="true" >
<alpha
android:duration="1100"
android:fromAlpha="1.0"
android:interpolator="@android:anim/accelerate_interpolator"
android:toAlpha="0.0" />
</set>
<!-- move_animation.xml -->
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:interpolator="@android:anim/linear_interpolator"
android:fillAfter="true">
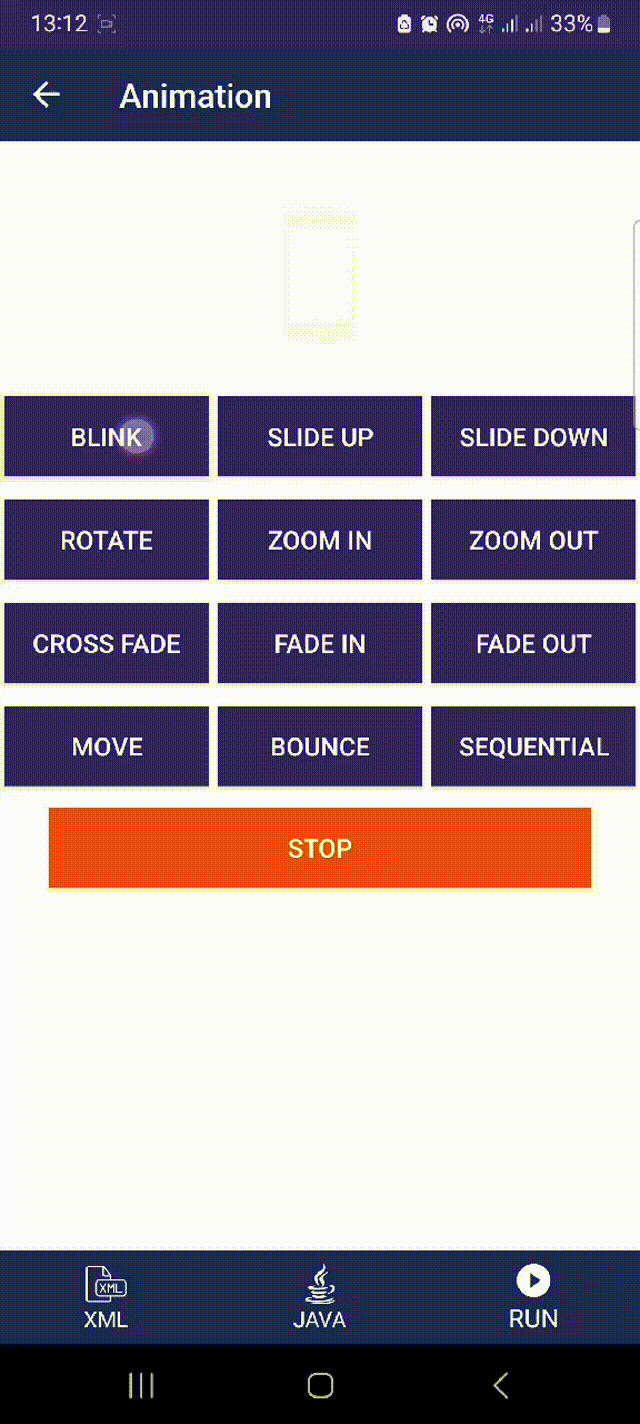